Okay, so today I wanna share my experience with “clinger stages.” Honestly, I stumbled upon this whole thing kinda by accident, but it turned out to be a real learning experience.
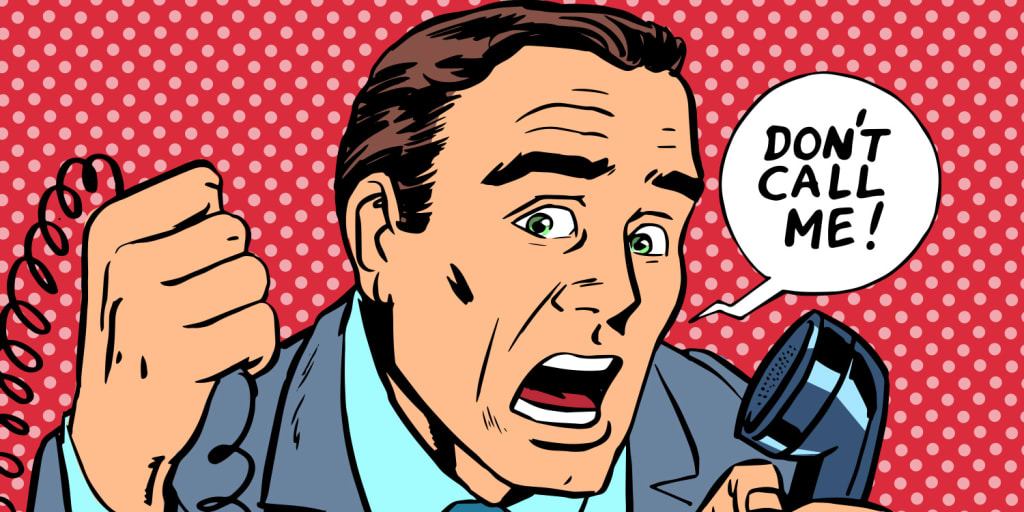
It all started when I was trying to figure out how to better manage a certain kind of…let’s call it “persistent connection” in one of my projects. Think of it like, you want something to keep trying to connect even if it fails the first few times. I was banging my head against the wall trying to get it right, using a simple loop and retry mechanism. It worked, kinda, but it was clunky and ate up resources like crazy.
So, I started digging around online, searching for better ways to handle this. That’s when I came across the idea of “clinger stages.” Initially, I was like, “What the heck is that?” It sounded like some weird relationship advice, not something useful for my coding.
But the more I read, the more it clicked. The basic idea is to break down the connection process into distinct stages, and then have a strategy for each stage. Instead of just blindly retrying, you can be smarter about it. Like, maybe the first few retries are really quick, and then you start slowing down as time goes on.
Here’s what I actually did:
- Stage 1: Immediate Retries. The goal here is to handle temporary glitches, like a network hiccup. I set up a loop that retried the connection every few milliseconds for the first second or two. This catches those quick, annoying problems.
- Stage 2: Gradual Backoff. If the immediate retries failed, I figured there was something more serious going on. So, I implemented a backoff strategy. The retry interval started at, say, 1 second, and then doubled with each failure, up to a maximum of maybe 30 seconds. This gives the system time to recover without constantly bombarding it with requests.
- Stage 3: Long-Term Polling. If even the backoff strategy didn’t work, I assumed the service was properly down. At this point, I switched to a really slow polling interval, like once every five minutes. This minimizes resource usage but still keeps an eye out for when the service comes back online.
- Stage 4: Giving Up (Temporarily). After a set amount of time (like an hour or two) of failing at Stage 3, I decided it was better to just log the issue and stop trying for a while. Maybe there’s a bigger problem that needs human intervention, or the service is just gone for good. Trying forever just wastes resources. But, crucially, I added a mechanism to automatically re-enable the clinger after a longer period, like overnight. This gives the system a fresh chance to connect when things might be different.
To implement this, I used a combination of timers, counters, and some simple state management. I basically had a function that would:
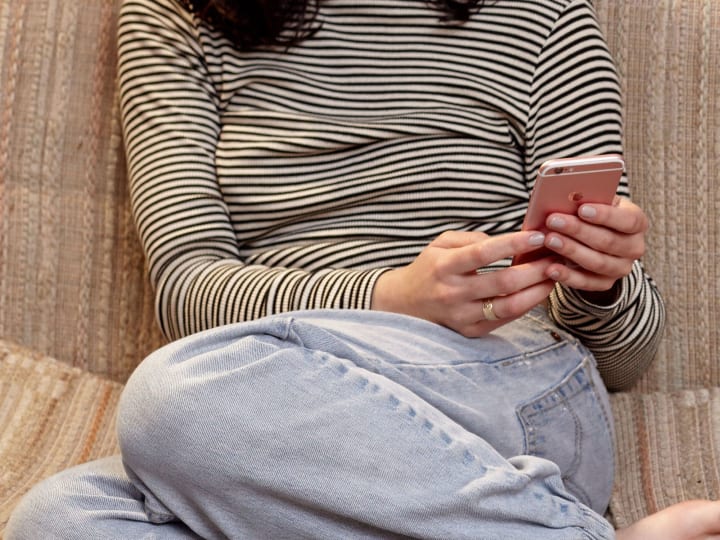
- Attempt to connect.
- If it failed, increment a retry counter.
- Based on the retry counter, determine the next retry interval using the staged strategy.
- Set a timer for that interval.
- When the timer expired, repeat from step 1.
The result was pretty awesome. The system became much more resilient to temporary outages. It also stopped hogging resources when things were really down. Plus, the staged approach gave me much finer control over how the connection process behaved.
Of course, it wasn’t all smooth sailing. I had to tweak the retry intervals and timeout values to find the sweet spot for my specific use case. And I had to add some logging to track the different stages and make sure everything was working as expected.
But overall, learning about “clinger stages” was a game-changer. It transformed a clunky, inefficient retry mechanism into something much more robust and well-behaved. If you’re dealing with persistent connections, I highly recommend giving it a try.