Alright, so today I wanna talk about something I was messing around with recently: relationship structures in my personal project. It wasn’t as straightforward as I initially thought, and I definitely learned a few things along the way.
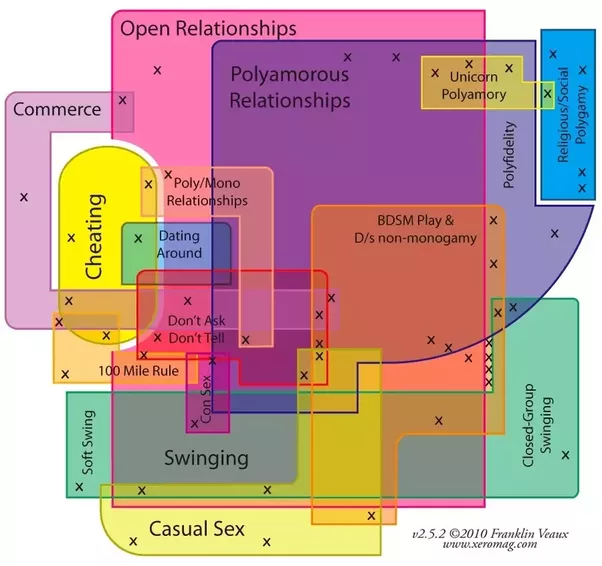
First off, I had this idea for a data model where different entities could relate to each other in various ways. Think of it like users, posts, comments – the usual suspects, but with some custom twists that made things…interesting.
Initially, I started just slapping foreign keys everywhere. “Okay, this comment belongs to this user,” bam, foreign key. “This post has these tags,” bam, another one. It worked, kinda, but it quickly became a nightmare to query. Imagine trying to fetch a user and all their associated posts, comments, likes, shares, etc. The queries were getting huge and slow.
Then I thought, “Maybe I need a better ORM.” I tried a couple different ones, but the underlying issue wasn’t the ORM itself; it was my terrible data structure. I was trying to force-fit complex relationships into a simple relational model.
So, I took a step back and started sketching things out on paper (yeah, old school). I really thought about the relationships: are they one-to-one, one-to-many, many-to-many? And more importantly, how are they many-to-many? That last part was key.
I realized I was missing a crucial abstraction: relationship types. Instead of just having a bunch of implicit relationships defined by foreign keys, I needed to explicitly define the type of relationship. Like, “this user follows this other user,” or “this post is authored by this user.”
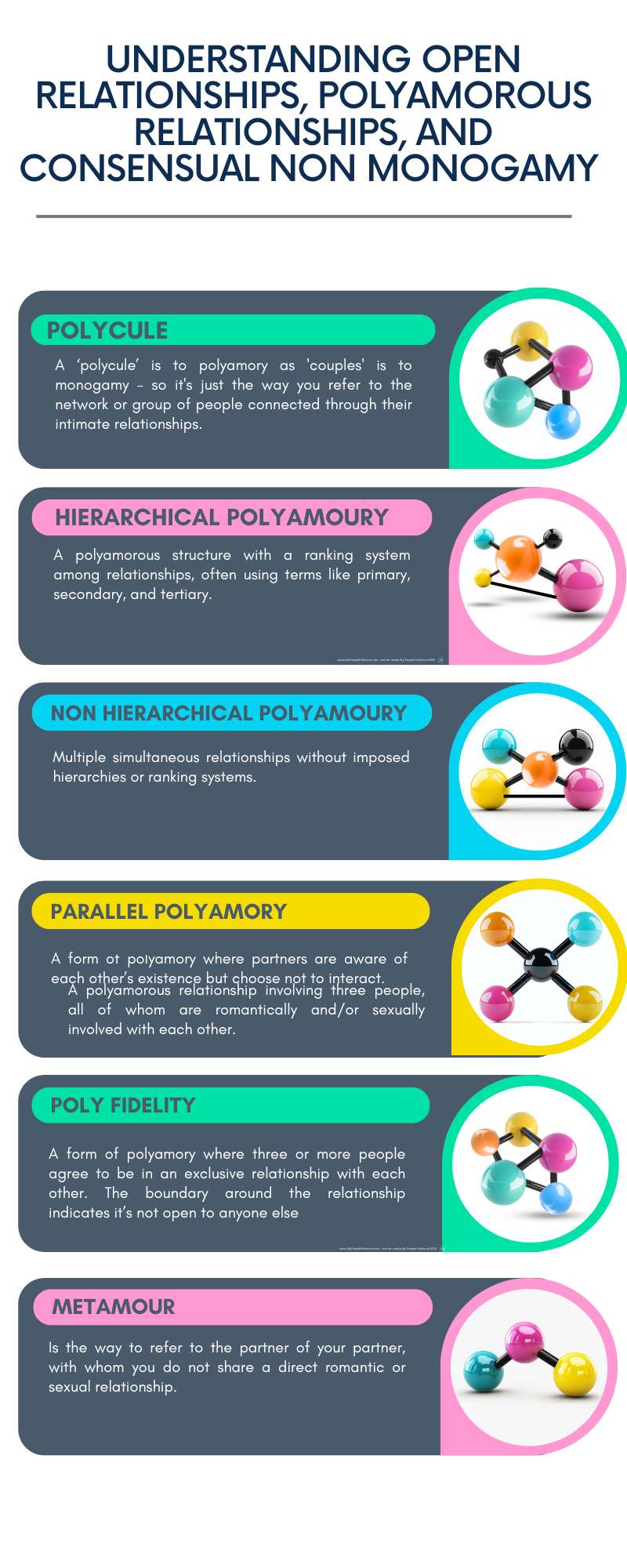
To implement this, I created a new table (let’s call it `relationships`) with columns like `source_id`, `target_id`, and `relationship_type`. The `source_id` and `target_id` would point to the related entities, and the `relationship_type` would be a string that defined the type of relationship (e.g., “follows”, “authored_by”, “likes”).
Here’s the tricky part: I needed a way to make this generic enough to work with any entity type. So, instead of having separate tables for users, posts, comments, etc., I used a single “entities” table with a `type` column to differentiate them (e.g., `type` = “user”, `type` = “post”). This meant that `source_id` and `target_id` in the `relationships` table would always point to rows in the `entities` table.
Yeah, it’s kinda like EAV (Entity-Attribute-Value), but for relationships instead of attributes. And yes, I know EAV gets a lot of hate, but in this specific case, it actually made things simpler.
Once I had this structure in place, querying became much easier. I could fetch all the entities related to a specific entity by querying the `relationships` table and filtering by `source_id` and `relationship_type`. I could also easily add new relationship types without having to modify the database schema.
Of course, there were some downsides. The queries became a bit more complex, and I had to be careful to ensure data consistency (e.g., preventing circular relationships). But overall, it was a huge improvement over the original foreign-key-everywhere approach.
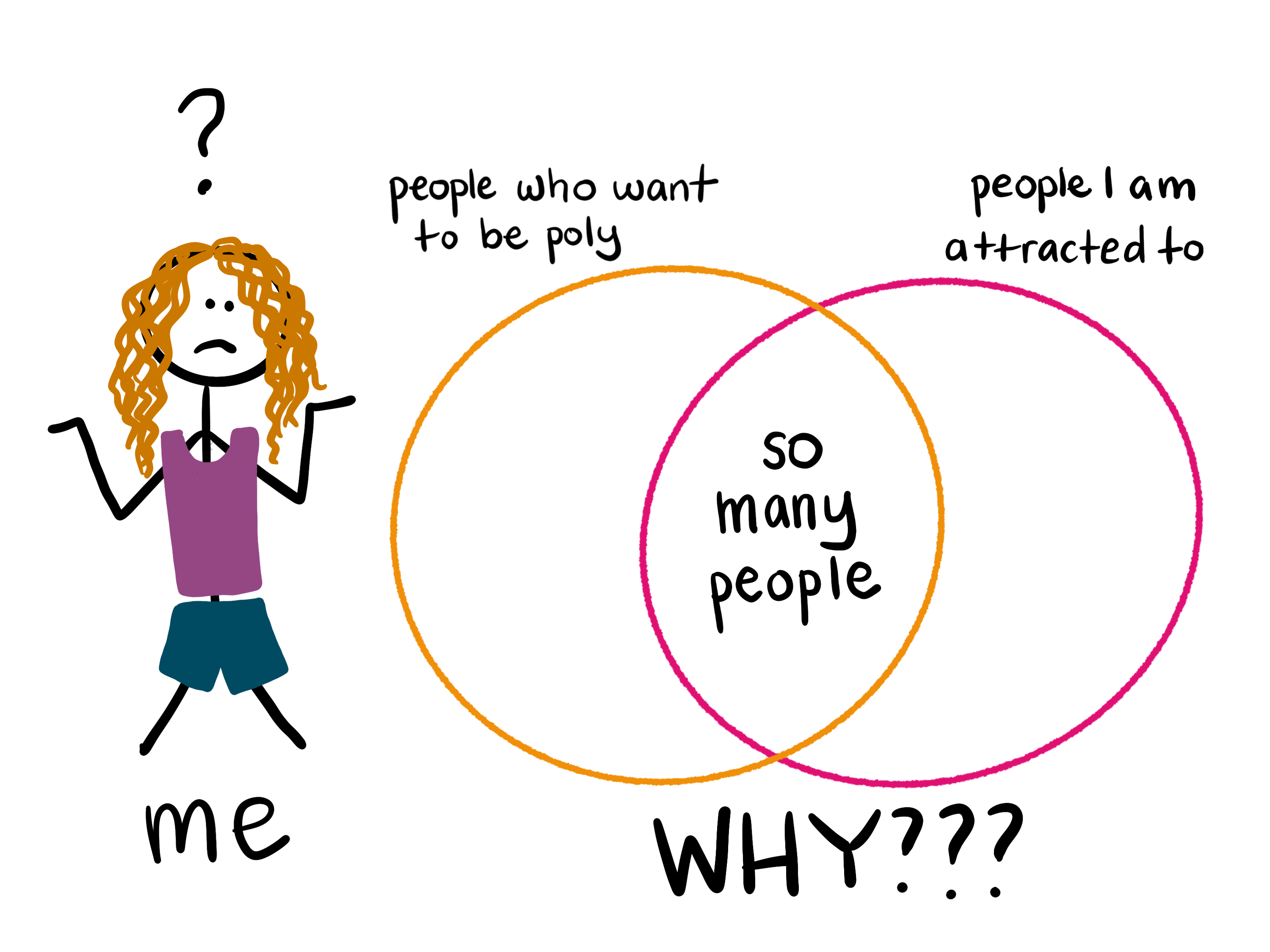
Key takeaways:
- Don’t be afraid to rethink your data structure if it’s not working.
- Explicitly defining relationship types can make complex relationships much easier to manage.
- Sometimes, unconventional data models (like EAV-ish) can be the right solution, even if they’re not the most popular.
This whole process was a bit of a rollercoaster, but I’m happy with where I ended up. It’s not perfect, but it’s a lot more flexible and maintainable than what I started with. And that’s what matters, right?
What I Learned
Here are some things I specifically learned while messing with this structure:
- Indexing is Your Friend: Make sure you have proper indexes on `source_id`, `target_id`, and `relationship_type` in the `relationships` table. Otherwise, your queries will be SLOW.
- Caching is Essential: Complex relationship queries can be expensive. Cache the results aggressively to avoid hitting the database repeatedly.
- Data Validation is Crucial: Implement robust data validation to prevent invalid relationships (e.g., circular relationships, relationships between entities of the wrong type).