Alright, so today I’m gonna walk you through this little thing I did called “letter from friend to friend.” It’s not rocket science, but I learned a thing or two, so figured I’d share.
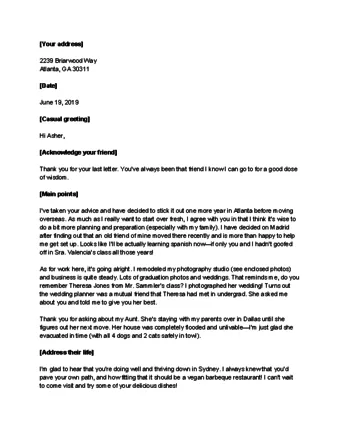
First off, I started with a basic idea: I wanted to create a simple program where two friends could “exchange” letters virtually. No fancy stuff, just plain text.
Then, I grabbed my trusty Python. Why Python? Because it’s quick and dirty, perfect for this kind of project. I fired up my IDE and created a new file called `letter_*`.
Next, I thought about the core functionality. We needed a way to:
- Write a letter
- Store the letter
- Retrieve the letter
- “Send” it to the other friend (simulate it)
So, I began coding. I defined a simple function called `write_letter()` that prompted the user to type their letter. It just used the `input()` function. Nothing special. Then, I made another function called `store_letter()`. This one took the letter as input and wrote it to a text file. I called it `*`. Super simple.
After that, I created `read_letter()`. This function opened `*` and read its content. Finally, I made a function called `send_letter()` which really just printed the content of the retrieved letter to the console. It’s a simulation, remember?
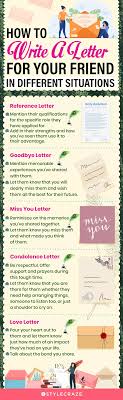
Now, the tricky part was putting it all together. I needed a main function to orchestrate everything. I created a menu with options like “Write Letter,” “Read Letter,” and “Send Letter.” I used a simple `while` loop to keep the program running until the user chose to exit.
I ran into a few snags, of course. Like, forgetting to close the file after writing to it. Silly mistakes, but that’s part of the process. I also had some encoding issues when I tried to use special characters in the letter. Had to mess around with `utf-8` encoding to fix that.
Once I ironed out the kinks, I tested it with a friend. We took turns writing and “sending” letters. It was pretty basic, but it worked! We even added a little bit of encryption (super basic Caesar cipher) just for fun.
In the end, it wasn’t anything groundbreaking, but I learned a few things about file handling, user input, and basic program flow. Plus, it was a fun little exercise in problem-solving. The code is probably terrible, but hey, it works! And that’s what matters, right?